<< Android-Note
シークバーから値を取得する
SeekBarシークバーはサム部分とプログレス部分の2つからなります。

上の画像でいうならタッチで移動できる丸い部分がサムで、水平バーがプログレス部分に当たります。
ここでは基本的なシークバーの使い方について紹介します。
レイアウトの作成
シークバーを作るにはSeekBarクラスを利用します。
ここでは次のようなレイアウトを作成しました。
<?xml version="1.0" encoding="utf-8" ?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<TextView
android:id="@+id/seek_bar_status_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="5dp"
android:text="@string/volume_control_dlg_msg"
android:textSize="15sp" />
<SeekBar
android:id="@+id/volume_ctr_seek"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="100" />
<TextView
android:id="@+id/show_volume_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="right" />
</LinearLayout>
このレイアウトはシークバーとその値を音量として表示するテキストビューとシークバーの状態を表示するテキストビューの3つから成り立っています。
シークバーの最大値は次のようにmaxプロパティに指定します。
android:max="100"
シークバーの値を取得する
次にコード中でシークバーの値と状態を取得し、テキストビューに表示するようにしてみます。
それが次のコードです。
public class MainActivity extends Activity { public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void onStart() { final TextView showSeekStatusText = (TextView)dlg_view.findViewById(R.id.seek_status_text); SeekBar volumeSeek = (SeekBar)dlg_view.findViewById(R.id.volume_ctr_seek); final TextView showVolumeText = (TextView)dlg_view.findViewById(R.id.show_volume_text); //シークバーを監視するリスナーを設定 volumeSeek.setOnSeekBarChangeListener( new SeekBar.OnSeekBarChangeListener() { @Override public void onStopTrackingTouch(SeekBar seekBar) { showSeekStatusText.setText("状態 : トラッキング開始"); } @Override public void onStartTrackingTouch(SeekBar seekBar) { showSeekStatusText.setText("状態 : トラッキング終了"); } @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { showSeekStatusText.setText("状態 : 変化中"); showVolumeText.setText("音量 " + progress); //シークバーの値を表示 } }); } }
OnSeekBarChangeListenerを使ってシークバーの監視をしています。
リスナー内の3つのメソッドはそれぞれ次の場合に呼ばれます。
- onStopTrackingTouch(SeekBar seekBar)
シークバーを動かしてから、シークバーをはなした場合に呼び出されます。
- onStartTrackingTouch(SeekBar seekBar)
シークバーに触れていない状態からシークバーに触れて動かす間に呼ばれます。
- onProgressChanged(SeekBar seekBar, int progress, boolean fromUser)
シークバーを動かしている最中に呼ばれます。progressがシークバーの値です。
このコードを動かしたときのシークバーの状態は次の画像のようになります。
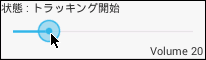
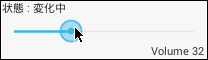
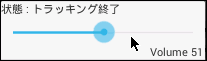
© Kaz