<< Android-Note
通知バーにアプリの通知を表示する方法
通知バーとはアプリ本体の上に位置する次のようなバーのことです。

左側にアプリの通知を表示することができます。
通知の作成
例えばあるアクティビティ(FirstActivity)から別のアクティビティ(SecondActivity)を起動する場合を考えます。
このとき、通知バーに起動するアクティビティの通知を表示するには次のようなコードを書きます。
public class FirstActivity extends Activity { public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button showNotificationButton = (Button)findViewById(R.id.show_ntf_btn); showNotificationButton.setOnClickListener(new View.onClickListener() { public void onClick(View v) { showNotification(); } }); } /*アクティビティを起動してアプリの通知を表示する。*/ int notification_id = 0; public void showNotification() { NotificationCompat.Builder ntfBuilder = new NotificationCompat.Builder(this) .setContentTitle("Mail") .setSmallIcon(R.drawable.mail) .setContentText("mail app"); /*通知バーに表示する通知のアイコンとタイトルと本文を設定*/ /*起動するインテント*/ Intent secondIntent = new Intent(this.getApplicationContext(), SecondActivity.class); secondIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); //新しいタスクで起動 /*FirstActivityのスタックに起動するアクティビティを追加*/ TaskStackBuilder stackBuilder = TaskStackBuilder.from(this); stackBuilder.addParentStack(FirstActivity.class); stackBuilder.addNextIntent(secondIntent); PendingIntent secondPendingIntent = stackBuilder.getPendingIntent( 0, PendingIntent.FLAG_UPDATE_CURRENT); ntfBuilder.setContentIntent(secondPendingIntent); NotificationManager ntfManager = (NotificationManager)getSystemService( Context.NOTIFICATION_SERVICE); ntfManager.notify(notification_id, ntfBuilder.getNotification()); //通知を表示する。 } }
ボタンを押すと新たにアプリの通知を表示するようにしています。
この例ではnotification_idをアプリの通知を識別するためのIDとしてつけています。
Notification.BuilderとTaskStackBuilderはAPIレベルが11以上必要なのでそれぞれ代わりにandroid.support.v4.appパッケージのNotificationCompatとTaskStackBuilderを使用します。
通知バーがスライドされると次のようなアイコンと画像と本文を持った通知が表示され、クリックすることで指定のアクティビティが起動します。
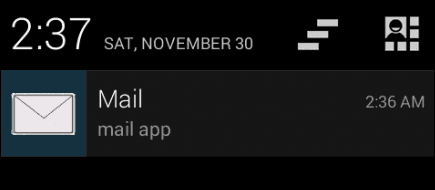
通知の削除
通知を削除したい場合は簡単で次の2行です。
NotificationManager ntf_manager = (NotificationManager)getSystemService(Context.NOTIFICATION_SERVICE);
ntf_manager.cancel(notification_id);
NotificationManagerを呼び出してからcancelメソッドで特定のID(ここではnotification_id)を持つ通知を削除することができます。
ちなみに通知が複数ある場合は次のようにcancelAllメソッドを呼び出せばすべての通知を削除できます。
NotificationManager ntf_manager = (NotificationManager)getSystemService(Context.NOTIFICATION_SERVICE);
ntf_manager.cancelAll();
関連項目
© Kaz